Plugins for the Equations
Description
BayesiaLab can include automatically functions defined by the user in its equation editor, to generate the conditional probability tables of the nodes. The defined functions can have a variable number of parameters. It is possible to interface Java, C, C++, FORTRAN, Mathematica, etc. This can be done directly through JNI or some specialized libraries. For more information and advice, you can contact Bayesia S.A.S.
Architecture
To allow this integration, a Java interface is included in the library BayesiaLab.jar located in the BayesiaLab's installation directory. To create its own function, the user must "implement" this interface with its own Java class. Once the class is created, a JAR library file must be created and copied into a directory named ext, which is a sub-directory of BayesiaLab's running directory.
If the created Java library needs additional files, they must be located in the ext sub-directory. Ensure the program is configured to look for the additional files in the ext directory.
For Windows, the path is:
C:\Documents and Settings\All Users\Application Data\BayesiaLab\ext
Interface
The Java interface that must be implemented is called ExternalFunction
and is located in the package com.Bayesia.BayesiaLab.ext
. Below is its content:
package com.Bayesia.BayesiaLab.ext;
public interface ExternalFunction {
public static final int REAL = 1;
public static final int INTEGER = 2;
public static final int BOOLEAN = 3;
public static final int STRING = 4;
public boolean initialization();
public boolean finalization();
public void beforeEvaluation(int combinationNumber, int sampleNumber);
public void afterEvaluation();
public void evaluate() throws ArithmeticException;
public String getName();
public String getDescription();
public boolean isVariableParameterNumber();
public void setUsedParameterNumber(int parameterNumber);
public int getParameterNumber();
public String getParameterNameAt(int parameterIndex);
public int getParameterTypeAt(int parameterIndex);
public int getReturnType();
public void setParameterValueAt(int parameterIndex, int value);
public void setParameterValueAt(int parameterIndex, double value);
public void setParameterValueAt(int parameterIndex, boolean value);
public void setParameterValueAt(int parameterIndex, String value);
public int getIntegerResult();
public double getRealResult();
public boolean getBooleanResult();
public String getStringResult();
}
Example of Implementation
The following example uses a Windows dynamic library (DLL) that returns the sum of two real numbers.
import com.Bayesia.BayesiaLab.ext.*;
public class Sum implements ExternalFunction {
static {
System.loadLibrary("ext/Sum");
}
private static final String[] parameters = {"a", "b"};
private static final int[] parameterTypes = {REAL, REAL};
private double[] values = new double[2];
private double result = Double.NaN;
private native double compute(double a, double b);
public void evaluate() throws ArithmeticException {
result = compute(values[0], values[1]);
}
public boolean initialization() {
return true;
}
public boolean finalization() {
return true;
}
public void beforeEvaluation(int combinationNumber, int sampleNumber) {}
public void afterEvaluation() {}
public String getName() {
return "Sum";
}
public String getDescription() {
return "Return the sum of the real numbers a and b.";
}
public boolean isVariableParameterNumber() {
return false;
}
public void setUsedParameterNumber(int parameterNumber) {}
public int getParameterNumber() {
return parameters.length;
}
public String getParameterNameAt(int parameterIndex) {
return parameters[parameterIndex];
}
public int getParameterTypeAt(int parameterIndex) {
return parameterTypes[parameterIndex];
}
public int getReturnType() {
return REAL;
}
public void setParameterValueAt(int parameterIndex, double value) {
values[parameterIndex] = value;
}
public int getIntegerResult() {
return 0;
}
public double getRealResult() {
return result;
}
public boolean getBooleanResult() {
return false;
}
public String getStringResult() {
return null;
}
}
Compilation
The command line used to compile the Java class is:
javac -cp "C:\Program Files\Bayesia\BayesiaLab\BayesiaLab.jar" Sum.java
It may be necessary to indicate the path to the javac.exe
program located in the bin
directory of the JDK. The Sum.class
file will be generated.
Generating the Header File for the C++ Library
In order for Java to call the corresponding function in C++, it is necessary to generate a C++ header file to include it in the library. The command line used to generate the .h
file is:
javah Sum
The Sum.h
file will be generated. Below is an example header file:
/* DO NOT EDIT THIS FILE - it is machine generated */
#include <jni.h>
/* Header for class Sum */
#ifndef _Included_Sum
#define _Included_Sum
#ifdef __cplusplus
extern "C" {
#endif
JNIEXPORT jdouble JNICALL Java_Sum_compute(JNIEnv *, jobject, jdouble, jdouble);
#ifdef __cplusplus
}
#endif
For more information on JNI (Java Native Interface), consult the internet.
JAR File Generation
Once the Sum.java
file is compiled into Sum.class
, create the JAR library file using the following command:
jar -cf Sum.jar Sum.class
The Sum.jar
file must be placed in the ext sub-directory of BayesiaLab's running directory. The corresponding Sum.dll
library must also be placed in the ext sub-directory.
Starting
After creating the plugin and restarting BayesiaLab, the plugin is loaded and available in the equation editor.
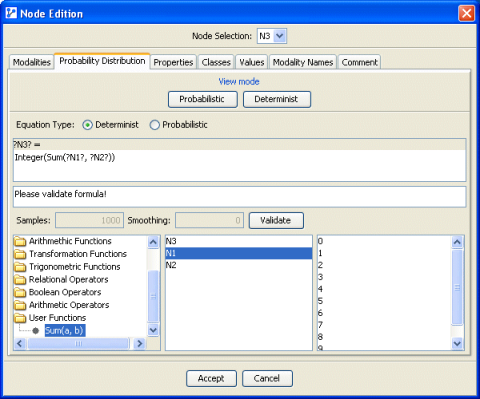